$rootScope is a parent object of all $scope angular objects created in a web page.
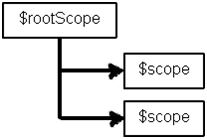
Let us understand how Angular does the same internally. Below is a simple Angular code which has multiple DIV tags and every tag is attached to a controller. So let us understand step by step how Angular will parse this and how the $rootScope and $scope hierarchy is created.
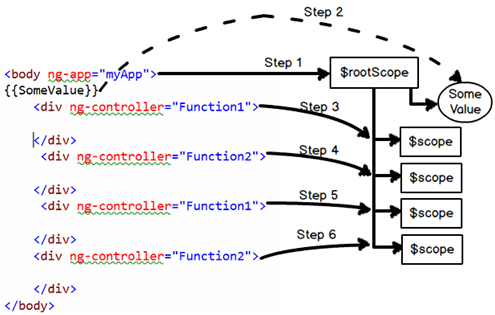
The Browser first loads the above HTML page and creates a DOM (Document Object Model) and Angular runs over the DOM. Below are the steps how Angular creates the rootscope and scope objects.
- Step 1: Angular parser first encounters the ng-app directive and creates a $rootScope object in memory.
- Step 2: Angular parser moves ahead and finds the expression {{SomeValue}}. It creates a variable.
- Step 3: Parser then finds the first DIV tag with ng-controller directive which is pointing to Function1 controller. Looking at the ng-controller directive, it creates a $scope object instance for Function1 controller. This object it then attaches to $rootScope object.
- Step 4: Step 3 is then repeated by the parser every time it finds a ng-controller directive tag. Step 5 and Step 6 is the repetition of Step 3.
If you want to test the above fundamentals, you can run the below sample Angular code. In the below sample code, we have created controllers Function1 and Function2. We have two counter variables one at the root scope level and other at the local controller level.
HTML
<script language=javascript>
function Function1($scope, $rootScope)
{
$rootScope.Counter = (($rootScope.Counter || 0) + 1);
$scope.Counter = $rootScope.Counter;
$scope.ControllerName = "Function1";
}
function Function2($scope, $rootScope)
{
$rootScope.Counter = (($rootScope.Counter || 0) + 1);
$scope.ControllerName = "Function2";
}
var app = angular.module("myApp", []); // creating a APP
app.controller("Function1", Function1); // Registering the VM
app.controller("Function2", Function2);
</script>
Below is the HTML code for the same. You can have attached Function1 and Function2 two times with ng-controller which means four instances will be created.
HTML
<body ng-app="myApp" id=1>
Global value is {{Counter}}<br />
<div ng-controller="Function1">
Child Instance of {{ControllerName}} created :- {{Counter}}
</div><br />
<div ng-controller="Function2">
Child Instance of {{ControllerName}} created :- {{Counter}}
</div><br />
<div ng-controller="Function1">
Child Instance of {{ControllerName}} created :- {{Counter}}
</div><br />
<div ng-controller="Function2">
Child Instance of {{ControllerName}} created :- {{Counter}}
</div><br />
</body>

Above is the output of the code you can see the global variable of root scope has be incremented four times because four instances of $scope have been created inside $rootScope object.