Promises are POST PROCESSING LOGICS which you want to execute after some operation / action is completed. While deferred helps to control how and when those promise logics will execute.
We can think about promises as WHAT
we want to fire after an operation is completed while deferred controls WHEN
and HOW
those promises will execute.
For example, after an operation is complete, you want to a send a mail, log in to log file and so on. So these operations you will define using promise. And these promise logics will be controlled by deferred.
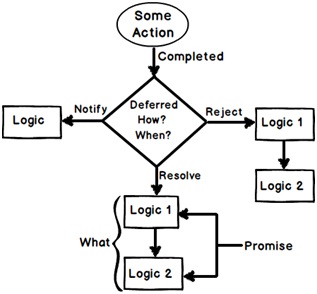
We are thankful to www.stepbystepschools.net for the above image.
So once some action completes, deferred gives a signal Resolve
, Reject
or Notify
and depending on what kind of signal is sent, the appropriate promise logic chain fires.
$q
is the angular service which provides promises and deferred functionality.
Using promises, deferred and q
service is a 4 step process:
- Step 1: Get the
q
service injected from Angular. - Step 2: Get deferred object from
q
service object. - Step 3: Get
Promise
object from deferred object. - Step 4: Add logics to the promise object.
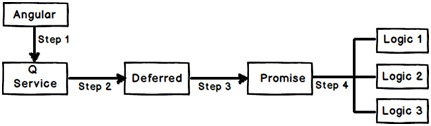
Below is the Angular code for the above four steps.
function SomeClass($scope,$q) {
var defer = $q.defer();
var promise = defer.promise;
promise.then(function () {
alert("Logic1 success");
}, function () {
alert("Logic 1 failure");
});
promise.then(function () {
alert("Logic 2 success");
}, function () {
alert("Logic 2 failure");
});
}
So now depending on situations, you can signal your promise logics via deferred to either fire the success events or the failure events.
defer.resolve();
defer.reject();